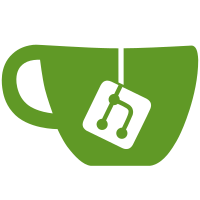
# Conflicts: # Blacksmith/UserSettings/Layouts/default-2022.dwlt # box1/Assets/Scenes/SampleScene.unity # box1/Assets/Script/UI/MainPanel.cs # box1/UserSettings/Layouts/default-2022.dwlt
536 lines
14 KiB
C#
536 lines
14 KiB
C#
using System;
|
||
using System.Collections;
|
||
using System.Collections.Generic;
|
||
using System.Linq;
|
||
using System.Reflection;
|
||
using TTSDK;
|
||
using UnityEditor;
|
||
using UnityEngine;
|
||
|
||
public static class DataManager
|
||
{
|
||
public static bool GetFirst()
|
||
{
|
||
return GetPrefab("First")==1;
|
||
}
|
||
|
||
public static void SaveFirst()
|
||
{
|
||
SetPrefab("First",1);
|
||
}
|
||
|
||
public static Dictionary<int, int> ItemDictionary
|
||
{
|
||
get
|
||
{
|
||
if (itemDictionary==null)
|
||
{
|
||
itemDictionary = (Dictionary<int, int>)LoadValue(typeof(Dictionary<int, int>), "ItemDic");
|
||
}
|
||
return itemDictionary;
|
||
}
|
||
set
|
||
{
|
||
BDebug.Log(value);
|
||
itemDictionary = value;
|
||
SaveValue("ItemDic", itemDictionary);
|
||
}
|
||
}
|
||
private static Dictionary<int, int> itemDictionary;
|
||
|
||
public static int GuidInt(int key)
|
||
{
|
||
return (int)LoadValue(typeof(int), "guidItem"+key);
|
||
}
|
||
|
||
public static void SaveGuidItem(int key,int num)
|
||
{
|
||
SaveValue("guidItem" + key, num);
|
||
}
|
||
public static int GetItem(int id)
|
||
{
|
||
return (ItemDictionary.ContainsKey(id) ? ItemDictionary[id] : 0)+GuidInt(id);
|
||
//return ItemDatas.FirstOrDefault(vaItemData => vaItemData.item.id == id);
|
||
}
|
||
|
||
public static void SaveItem(int key, int num)
|
||
{
|
||
if (key==0)
|
||
{
|
||
return;
|
||
}
|
||
|
||
if (key==1)
|
||
{
|
||
if (num > 30)
|
||
{
|
||
num = 30;
|
||
}
|
||
}
|
||
ItemDictionary[key] = num;
|
||
ItemDictionary = ItemDictionary;
|
||
}
|
||
|
||
public static void SetTime(string key)
|
||
{
|
||
var ts = GetTimeStamp(DateTime.Now.ToUniversalTime());
|
||
SaveValue("time" + key, ts);
|
||
}
|
||
|
||
//获取时间戳
|
||
private static int GetTimeStamp(DateTime dt)
|
||
{
|
||
TimeSpan ts = dt - new DateTime(1970, 1, 1, 0, 0, 0);
|
||
return (int)System.Convert.ToInt64(ts.TotalSeconds);
|
||
}
|
||
|
||
public static void SetDStore(int id,int num)
|
||
{
|
||
SetPrefab("storeDay"+id.ToString(),num);
|
||
}
|
||
public static int GetDStore(int id)
|
||
{
|
||
return GetPrefab("storeDay"+id.ToString());
|
||
}
|
||
|
||
public static void SetDDiscount(int id,int dis)
|
||
{
|
||
SetPrefab("storeDiscount"+id.ToString(),dis);
|
||
}
|
||
public static int GetDDiscount(int id)
|
||
{
|
||
return GetPrefab("storeDiscount"+id.ToString());
|
||
}
|
||
//时间戳换算成时间
|
||
private static DateTime ConvertIntDatetime(Int64 utc)
|
||
{
|
||
System.DateTime startTime = TimeZone.CurrentTimeZone.ToLocalTime(new System.DateTime(1970, 1, 1,0,0,0));
|
||
startTime = startTime.AddSeconds(utc);
|
||
return startTime;
|
||
}
|
||
public static bool NextDay(string key)
|
||
{
|
||
var testTime2 =GetTimeStamp (DateTime.Now);
|
||
var testTime = GetTimeStamp(GetTime(key));
|
||
var testTimeLingchen = testTime - ((testTime + 8 * 3600) % 86400);
|
||
if (testTime2 > testTime)
|
||
{
|
||
if (testTime2 - testTimeLingchen < 24 * 60 * 60)
|
||
{
|
||
BDebug.Log("进入时间 和 上次进入时间,在同一天,且在上次进入时间之后");
|
||
return false;
|
||
}
|
||
else
|
||
{
|
||
BDebug.Log("进入时间 和 上次进入时间,不同一天,且在上次进入时间之后");
|
||
return true;
|
||
}
|
||
}
|
||
else if (testTime2 < testTime)
|
||
{
|
||
if (testTime2 - testTimeLingchen < 24 * 60 * 60)
|
||
{
|
||
BDebug.Log("进入时间 和 上次进入时间,在同一天,且在上次进入时间之前");
|
||
return false;
|
||
}
|
||
else
|
||
{
|
||
BDebug.Log("进入时间 和 上次进入时间,不同一天,且在上次进入时间之前");
|
||
return false;
|
||
}
|
||
}
|
||
else
|
||
{
|
||
BDebug.Log("进入时间 和 上次进入时间,是同一个时刻");
|
||
return false;
|
||
}
|
||
}
|
||
public static DateTime GetTime(string key)
|
||
{
|
||
return ConvertIntDatetime(GetPrefab("time" + key));;
|
||
}
|
||
|
||
public static int GetMinute(string key)
|
||
{
|
||
TimeSpan timeSpan = new TimeSpan(DateTime.Now.Ticks - GetTime(key).Ticks);
|
||
return timeSpan.Minutes;
|
||
}
|
||
|
||
public static int GetHours(string key)
|
||
{
|
||
//计算两个时间间隔
|
||
TimeSpan timeSpan = new TimeSpan(DateTime.Now.Ticks - GetTime(key).Ticks);
|
||
return timeSpan.Hours;
|
||
}
|
||
|
||
public static int GetDay(string key)
|
||
{
|
||
//计算两个时间间隔
|
||
TimeSpan timeSpan = new TimeSpan(DateTime.Now.Ticks - GetTime(key).Ticks);
|
||
return timeSpan.Days;
|
||
}
|
||
|
||
public static int GetPhysical()
|
||
{
|
||
AddPhysical();
|
||
return GetItem(1);
|
||
}
|
||
|
||
public static void RemovePhysical()
|
||
{
|
||
RemoveItem(1,5);
|
||
}
|
||
|
||
public static void AddPhysical()
|
||
{
|
||
var p = (float)GetMinute("physical")/10;
|
||
if (p>1)
|
||
{
|
||
NewPhysicalEvent();
|
||
SetPhysical((int)p);
|
||
}
|
||
}
|
||
public static void NewPhysicalEvent()
|
||
{
|
||
SetTime("physical");
|
||
}
|
||
public static void SetPhysical(int i)
|
||
{
|
||
var num = GetItem(1) + i;
|
||
if (num>30)
|
||
{
|
||
num = 30;
|
||
}
|
||
|
||
SaveItem(1, num);
|
||
}
|
||
public static void RemovePhysical(int num)
|
||
{
|
||
NewPhysicalEvent();
|
||
var p = GetItem(1) - num;
|
||
SaveItem(1 ,p);
|
||
}
|
||
/// <summary>
|
||
/// 获取当前图纸等级
|
||
/// </summary>
|
||
/// <param name="id"></param>
|
||
/// <param name="levelIDd"></param>
|
||
public static void SaveDrawLevel(int id,int levelIDd)
|
||
{
|
||
SaveValue("Drawing"+id.ToString(), levelIDd);
|
||
}
|
||
|
||
public static int GetDrawLevel(int id)
|
||
{
|
||
return GetPrefab("Drawing" + id.ToString());
|
||
}
|
||
|
||
public static void SaveDrawConsumables(int id,int num)
|
||
{
|
||
SaveValue("DrawNum"+id,num);
|
||
}
|
||
/// <summary>
|
||
/// 图鉴消耗进度
|
||
/// </summary>
|
||
/// <param name="id"></param>
|
||
/// <returns></returns>
|
||
public static int GetDrawConsumables(int id)
|
||
{
|
||
return GetPrefab("DrawNum"+id);
|
||
}
|
||
|
||
public static void SetNowLevel(int level)
|
||
{
|
||
SaveValue("Level", level);
|
||
}
|
||
public static int GetNowLevel()
|
||
{
|
||
return GetPrefab("Level");
|
||
}
|
||
|
||
public static void SetLevelLock(int level,bool bo)
|
||
{
|
||
SaveValue("LevelLock"+level,bo);
|
||
}
|
||
|
||
public static bool GetLevelLock(int level)
|
||
{
|
||
return (bool)LoadValue(typeof(bool), "LevelLock" + level);
|
||
}
|
||
public static void SaveItemUnLock(int key,bool bo)
|
||
{
|
||
SetPrefab("item-"+key,bo?1:0);
|
||
}
|
||
|
||
public static bool GetItemUnLock(int key)
|
||
{
|
||
return GetPrefab("item-"+key)==1;
|
||
}
|
||
|
||
public static void AddItem(int id, int itemNum)
|
||
{
|
||
SaveItem(id, GetItem(id) + itemNum);
|
||
// if (ItemDatas==null)
|
||
// {
|
||
// ItemDatas = new List<ItemData>();
|
||
// }
|
||
// var listData = ItemDatas.FirstOrDefault(vaItemData => vaItemData.item.id == itemData.id);
|
||
// if (listData==null)
|
||
// {
|
||
// ItemData saveData = new ItemData(itemData, itemNum);
|
||
// ItemDatas.Add(saveData);
|
||
// }
|
||
// else
|
||
// {
|
||
// listData.num += itemNum;
|
||
// }
|
||
}
|
||
|
||
public static void RemoveItem(int id, int num, Action<bool> action = null)
|
||
{
|
||
if (GetItem(id)>=num)
|
||
{
|
||
SaveItem(id,GetItem(id)-num);
|
||
action?.Invoke(true);
|
||
}
|
||
else
|
||
{
|
||
action?.Invoke(false);
|
||
}
|
||
ResetData();
|
||
}
|
||
public static void RemoveItem(int id,Action<bool> action)
|
||
{
|
||
if (GetItem(id)>=1)
|
||
{
|
||
SaveItem(id,GetItem(id)-1);
|
||
action.Invoke(true);
|
||
}
|
||
else
|
||
{
|
||
action.Invoke(false);
|
||
}
|
||
ResetData();
|
||
}
|
||
|
||
public static void RemoveItem(List<ItemDataNum> itemDatas,Action<bool> action)
|
||
{
|
||
foreach (var data in itemDatas)
|
||
{
|
||
if (GetItem(data.id)<data.num)
|
||
{
|
||
action.Invoke(false);
|
||
return;
|
||
}
|
||
}
|
||
|
||
foreach (var data in itemDatas)
|
||
{
|
||
SaveItem(data.id,GetItem(data.id)-data.num);
|
||
}
|
||
action.Invoke(true);
|
||
ResetData();
|
||
}
|
||
|
||
private static void ResetData()
|
||
{
|
||
|
||
}
|
||
|
||
|
||
public static void SetPrefab(string key ,int num)
|
||
{
|
||
|
||
SaveValue(key,num);
|
||
}
|
||
|
||
public static int GetPrefab(string key)
|
||
{
|
||
return (int)LoadValue(typeof(int),key);
|
||
}
|
||
//存储数据值
|
||
private static void SaveValue(string keyName,object value)
|
||
{
|
||
Type valueType = value.GetType();
|
||
//int类型存储
|
||
if (valueType == typeof(int))
|
||
{
|
||
//转换为string存储(正常按int存储时,类结构下的list会有数据读取错误-估计是unity的bug)
|
||
int v =(int)value;
|
||
TT.PlayerPrefs.SetString(keyName+"tostr", v.ToString());
|
||
|
||
// //正常int存储
|
||
// TT.PlayerPrefs.SetInt(keyName, v);
|
||
|
||
}
|
||
//float类型存储
|
||
else if (valueType == typeof(float))
|
||
{
|
||
TT.PlayerPrefs.SetFloat(keyName, (float)value);
|
||
}
|
||
//string类型存储
|
||
else if (valueType == typeof(string))
|
||
{
|
||
TT.PlayerPrefs.SetString(keyName, (string)value);
|
||
}
|
||
//bool类型存储,转为Int类型
|
||
else if (valueType == typeof(bool))
|
||
{
|
||
TT.PlayerPrefs.SetInt(keyName, (bool)value ? 1 : 0);
|
||
}
|
||
//List列表存储
|
||
else if (typeof(IList).IsAssignableFrom(valueType))
|
||
{
|
||
IList list = value as IList;
|
||
//先存储数量
|
||
TT.PlayerPrefs.SetInt(keyName, list.Count);
|
||
//再存储子项
|
||
for (int i = 0; i < list.Count; i++)
|
||
{
|
||
SaveValue( keyName + i,list[i]);
|
||
}
|
||
}
|
||
//Dictionary字典存储
|
||
else if (typeof(IDictionary).IsAssignableFrom(valueType))
|
||
{
|
||
IDictionary dic = value as IDictionary;
|
||
//先存储数量
|
||
TT.PlayerPrefs.SetInt(keyName, dic.Count);
|
||
//再存储子项
|
||
int i = 0;
|
||
foreach (var item in dic.Keys)
|
||
{
|
||
SaveValue( keyName + "_key_" + i,item);
|
||
SaveValue( keyName + "_value_" + i,dic[item]);
|
||
i++;
|
||
}
|
||
|
||
}
|
||
//自定义类型,需换取成员变量
|
||
else
|
||
{
|
||
//获取Type
|
||
Type dataType = value.GetType();
|
||
//获取成员变量
|
||
FieldInfo[] fields = dataType.GetFields();
|
||
//存储数据,命名规则:keyName_数据类型_字段类型_字段名
|
||
foreach (var item in fields)
|
||
{
|
||
// string name = keyName + "_" + dataType.Name + "_" + item.FieldType.Name + "_" + item.Name;
|
||
string name = keyName + "_" + item.Name;
|
||
SaveValue(name,item.GetValue(value));
|
||
}
|
||
}
|
||
//同步数据
|
||
TT.PlayerPrefs.Save();
|
||
}
|
||
//读取数据值
|
||
private static object LoadValue(Type type , string keyName)
|
||
{
|
||
//获取int
|
||
if (type == typeof(int))
|
||
{
|
||
//转换存储的string-获取int
|
||
string v = TT.PlayerPrefs.GetString(keyName+"tostr", "0");
|
||
return Convert.ToInt32(v);
|
||
|
||
//正常获取int
|
||
// return PlayerPrefs.GetInt(keyName, 0);
|
||
|
||
}
|
||
//获取float
|
||
else if (type == typeof(float))
|
||
{
|
||
return TT.PlayerPrefs.GetFloat(keyName, 0);
|
||
}
|
||
//获取string
|
||
else if (type == typeof(string))
|
||
{
|
||
return TT.PlayerPrefs.GetString(keyName, "");
|
||
}
|
||
//获取bool
|
||
else if (type == typeof(bool))
|
||
{
|
||
return TT.PlayerPrefs.GetInt(keyName, 0) == 1;
|
||
}
|
||
//获取List
|
||
else if (typeof(IList).IsAssignableFrom(type))
|
||
{
|
||
//获取长度
|
||
int count = TT.PlayerPrefs.GetInt(keyName, 0);
|
||
// //实例化list
|
||
|
||
IList list = Activator.CreateInstance(type) as IList;
|
||
for (int i = 0; i < count; i++)
|
||
{
|
||
//获取对应的子项数据,放入列表
|
||
list.Add(LoadValue(type.GetGenericArguments()[0], keyName + i));
|
||
}
|
||
return list;
|
||
}
|
||
//获取Dictinary字典
|
||
else if (typeof(IDictionary).IsAssignableFrom(type))
|
||
{
|
||
//获取长度
|
||
int count = TT.PlayerPrefs.GetInt(keyName, 0);
|
||
//实例化Dictionary
|
||
IDictionary dic = Activator.CreateInstance(type) as IDictionary;
|
||
for (int i = 0; i < count; i++)
|
||
{
|
||
//获取对应的子项
|
||
dic.Add(LoadValue(type.GetGenericArguments()[0], keyName + "_key_" + i), LoadValue(type.GetGenericArguments()[1], keyName + "_value_" + i));
|
||
}
|
||
return dic;
|
||
}
|
||
//获取其他类型
|
||
else
|
||
{
|
||
//实例化对象
|
||
object data = Activator.CreateInstance(type);
|
||
//获取成员变量
|
||
FieldInfo[] fields = type.GetFields();
|
||
//根据成员变量获取对应名称,keyname_类名_成员类型_成员名
|
||
for (int i = 0; i < fields.Length; i++)
|
||
{
|
||
FieldInfo info = fields[i];
|
||
// string name = keyName + "_" + type.Name + "_" + info.FieldType.Name + "_" + info.Name;
|
||
string name = keyName + "_" + info.Name;
|
||
//读取数据,设置给data对象
|
||
info.SetValue(data, LoadValue(info.FieldType, name));
|
||
}
|
||
return data;
|
||
}
|
||
}
|
||
|
||
}
|
||
[Serializable]
|
||
public class ItemDataNum
|
||
{
|
||
public int id;
|
||
public int num;
|
||
|
||
public ItemDataNum(int _id,int dataNum)
|
||
{
|
||
id = _id;
|
||
num = dataNum;
|
||
}
|
||
}
|
||
[Serializable]
|
||
public class Item
|
||
{
|
||
public int id;
|
||
}
|
||
|
||
[Serializable]
|
||
public class Consumables
|
||
{
|
||
public int levelID;
|
||
public int upgradID;
|
||
public int unm;
|
||
}
|
||
|
||
public class StoreData
|
||
{
|
||
public int id;
|
||
public int dic;
|
||
} |